Counting objects: 10, done. Delta compression using up to 4 threads. Compressing objects: 100% (10/10),…
How to use google analytics api in php
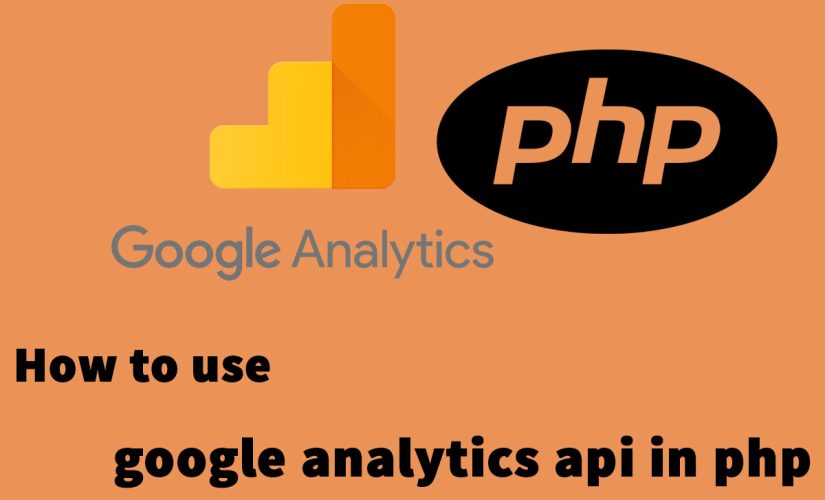
Google provides powerful API to get report from Google Analytics in your customer dashboard. After you integrate Google Analytics API, you can see real time activity of user who are on your site.
This tutorial will walk through the steps that require to access Google Analytics account, query the Analytics APIs, handle the API responses, and output the results. This tutorial use Core Reporting API v3.0, Management API v3.0, and OAuth2.0.
Step 1: Enable the Analytics API
First you need to use the setup tool, which guides you through creating a project in the Google API Console, enabling the API, and creating credentials. You will see the screenshot below after clicking on link above:

After you click on “Continue”, you will see the screenshot below:

Create a client ID
Open the Service accounts page. If prompted, select a project.

Click add Create Service Account, enter a name and description for the service account. You can use the default service account ID, or choose a different, unique one. When done click Create.


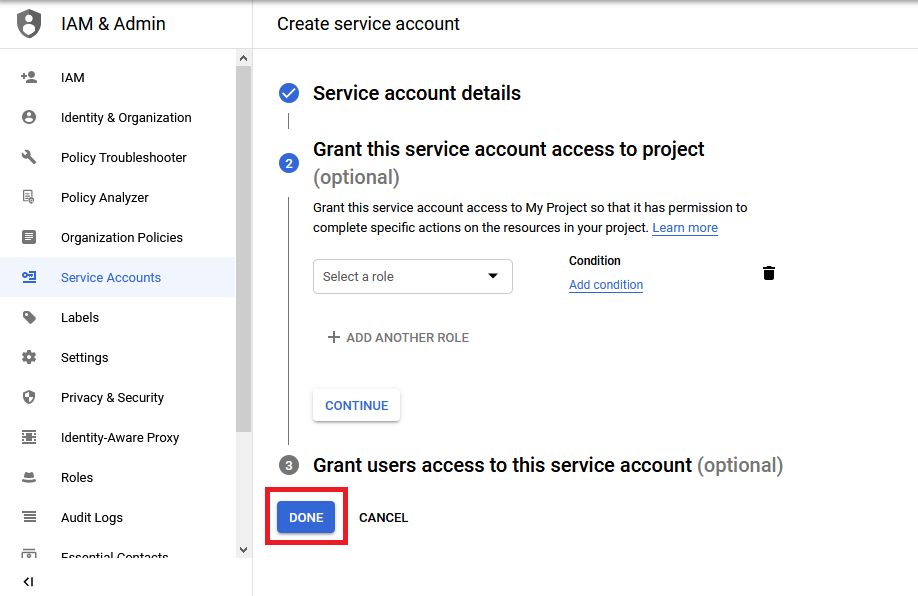
The Service account permissions (optional) section that follows is not required. Click Done. You will get the screenshot below after clink Done.
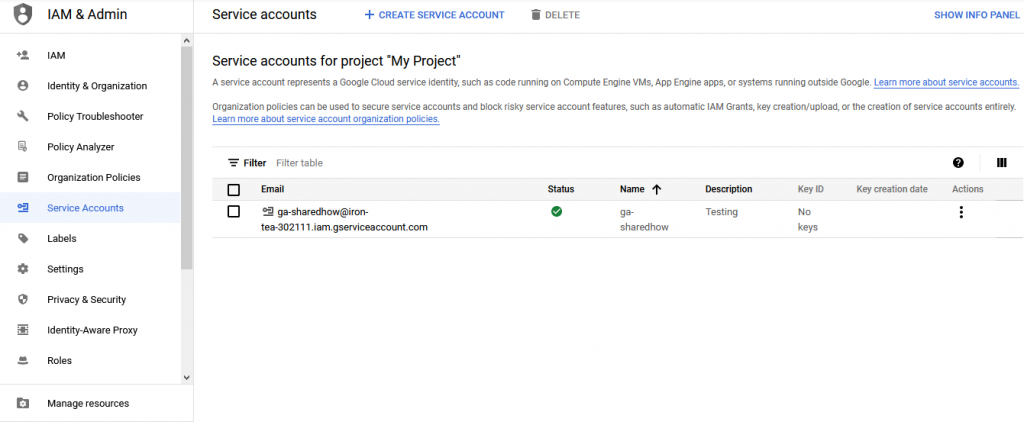
On the right side. Click add Create key.

In the pop-up that appears, select the format for your key: JSON is recommended.
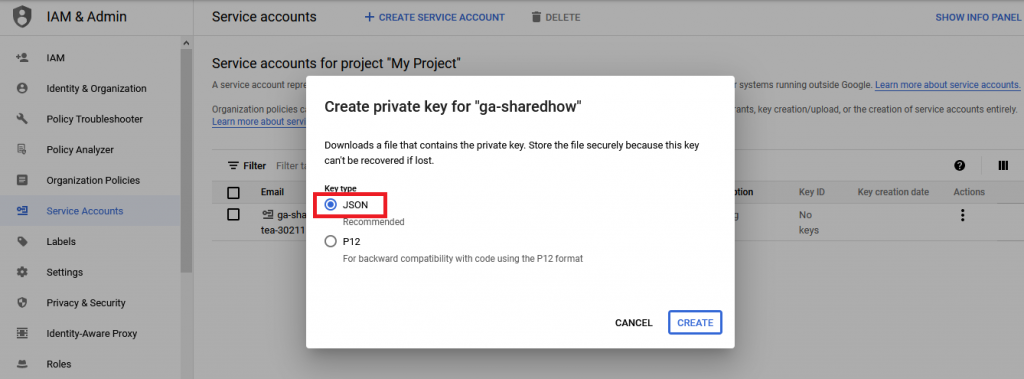
Click Create. Your new public/private key pair is generated and downloaded to your machine; it serves as the only copy of this key. For information on how to store it securely, see Managing service account keys.
Click Close on the Private key saved to your computer dialog to return to the table of your service accounts.

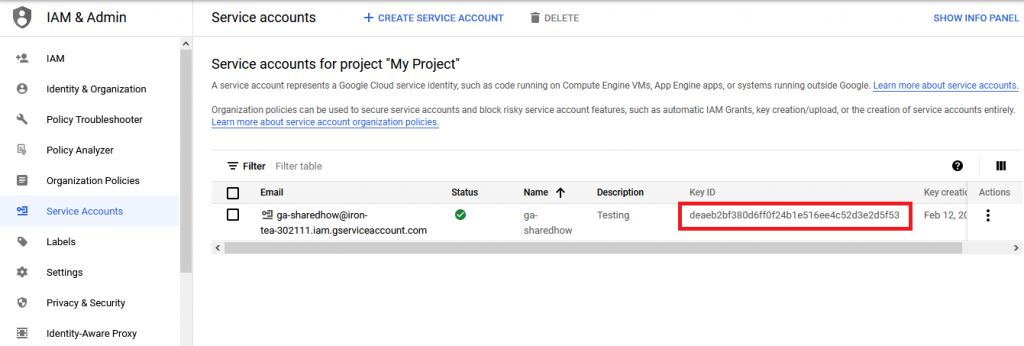
Add service account to Google Analytics account
In the file that you have saved into your computer, you will get the email of service account.

Use this email address to add a user to the Google analytics account you want to access via the API.
Step 2: Install the Google Client Library
You can get the Google APIs Client Library for PHP downloading the release or using Composer to install by using command below:
composer require google/apiclient:^2.0
Step 3: Setup the sample
To test if Google Analytics API work properly, you need to create a single file named HelloAnalytics.php
, which will contain the sample code below.
Copy or download the following source code to HelloAnalytics.php
.
Move the previously downloaded service-account-credentials.json
within the same directory as the sample code.
HelloAnalytics.php
<?php
// Load the Google API PHP Client Library.
require_once __DIR__ . '/vendor/autoload.php';
$analytics = initializeAnalytics();
$profile = getFirstProfileId($analytics);
$results = getResults($analytics, $profile);
printResults($results);
function initializeAnalytics()
{
// Creates and returns the Analytics Reporting service object.
// Use the developers console and download your service account
// credentials in JSON format. Place them in this directory or
// change the key file location if necessary.
$KEY_FILE_LOCATION = __DIR__ . '/service-account-credentials.json';
// Create and configure a new client object.
$client = new Google_Client();
$client->setApplicationName("Hello Analytics Reporting");
$client->setAuthConfig($KEY_FILE_LOCATION);
$client->setScopes(['https://www.googleapis.com/auth/analytics.readonly']);
$analytics = new Google_Service_Analytics($client);
return $analytics;
}
function getFirstProfileId($analytics) {
// Get the user's first view (profile) ID.
// Get the list of accounts for the authorized user.
$accounts = $analytics->management_accounts->listManagementAccounts();
if (count($accounts->getItems()) > 0) {
$items = $accounts->getItems();
$firstAccountId = $items[0]->getId();
// Get the list of properties for the authorized user.
$properties = $analytics->management_webproperties
->listManagementWebproperties($firstAccountId);
if (count($properties->getItems()) > 0) {
$items = $properties->getItems();
$firstPropertyId = $items[0]->getId();
// Get the list of views (profiles) for the authorized user.
$profiles = $analytics->management_profiles
->listManagementProfiles($firstAccountId, $firstPropertyId);
if (count($profiles->getItems()) > 0) {
$items = $profiles->getItems();
// Return the first view (profile) ID.
return $items[0]->getId();
} else {
throw new Exception('No views (profiles) found for this user.');
}
} else {
throw new Exception('No properties found for this user.');
}
} else {
throw new Exception('No accounts found for this user.');
}
}
function getResults($analytics, $profileId) {
// Calls the Core Reporting API and queries for the number of sessions
// for the last seven days.
return $analytics->data_ga->get(
'ga:' . $profileId,
'7daysAgo',
'today',
'ga:sessions');
}
function printResults($results) {
// Parses the response from the Core Reporting API and prints
// the profile name and total sessions.
if (count($results->getRows()) > 0) {
// Get the profile name.
$profileName = $results->getProfileInfo()->getProfileName();
// Get the entry for the first entry in the first row.
$rows = $results->getRows();
$sessions = $rows[0][0];
// Print the results.
print "First view (profile) found: $profileName\n";
print "Total sessions: $sessions\n";
} else {
print "No results found.\n";
}
}
If you setup project correctly, you will have the structure project like screenshot below:
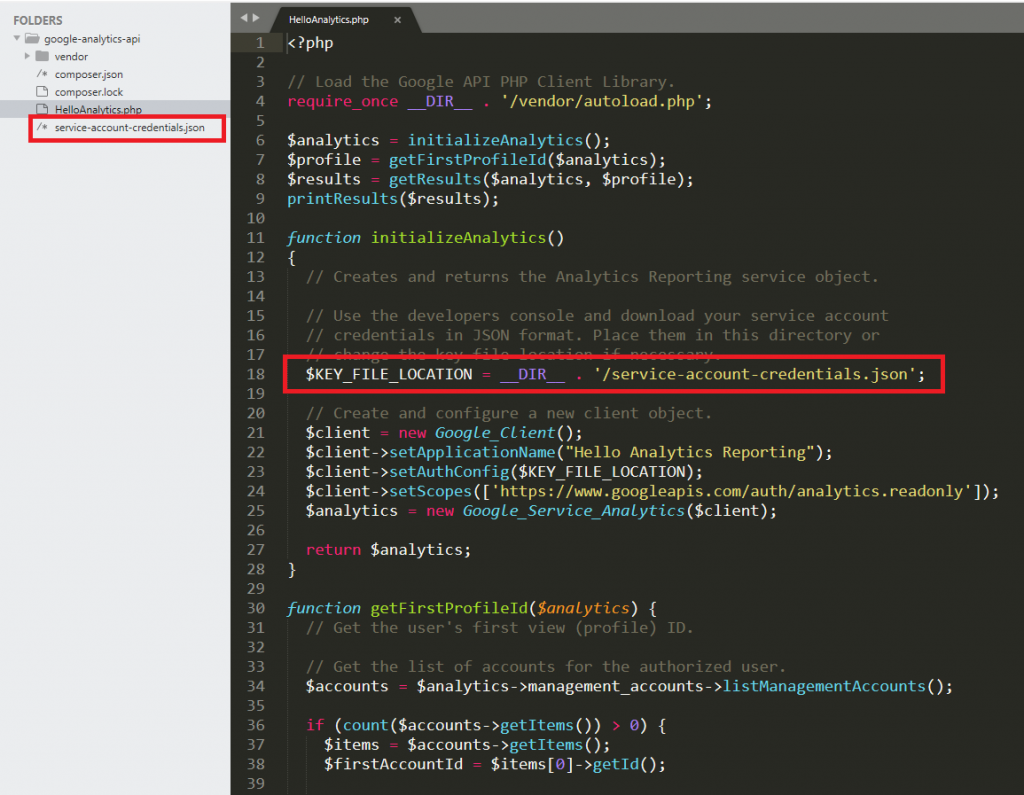
Step 4: Run the sample
After you enabled the Analytics API, installed the Google APIs client library for PHP, and set up the sample source code, the sample is ready to run.
php HelloAnalytics.php
You will get outputs like screenshot below after you run command above:

The sample outputs the name of the authorized user’s first Google Analytics view (profile) and the number of sessions for the last seven days.
Note: To successfully run the sample above you need to have at least one Google Analytics property and view (profile).
Source:
https://developers.google.com/analytics/devguides/config/mgmt/v3/quickstart/service-php
This Post Has 0 Comments