How to setup module in codeigniter

To create reusable code, CodeIgniter has an extension that enables the use of the Hierarchical Model View Controller (HMVC) pattern and make the CodeIgniter PHP Framework modular. Modules are groups of independent component that are grouped in their own folder and can have their own controller, model, view, library, config, helper and language files. This allows easy to distribute the independent component (MVC) in a single directory across the other CodeIgniter application.
Configuring HMVC Modules
Download the file from github https://github.com/jenssegers/codeigniter-hmvc-modules and place them into their corresponding folders in the application directory.

Open <project root directory>/application/config.php and add the location of your modules directory to the main config.php file:
/*
|--------------------------------------------------------------------------
| Modules locations
|--------------------------------------------------------------------------
|
| These are the folders where your modules are located. You may define an
| absolute path to the location or a relative path starting from the root
| directory.
|
*/
$config['modules_locations'] = array(APPPATH . 'modules/');
This is the basic structure of a HMVC module:
/modules
/signin
/controllers
/config
/helpers
/language
/libraries
/models
For module that we created, we can load its own resources like you always do. This is the sample of module signin:
Sample code of controller Signin.php
<?php
if (!defined('BASEPATH'))
exit('No direct script access allowed');
/**
* Description of signin
*
* @author https://www.sharedhow.com
*/
class Signin extends CI_Controller {
function __construct() {
parent::__construct();
if (version_compare(CI_VERSION, '2.1.0', '<')) {
$this->load->library('security');
}
$this->load->library('form_validation');
$this->load->helper('url');
}
function index() {
$data['title'] = 'Signin';
$this->form_validation->set_rules('username', 'Username', 'required');
$this->form_validation->set_rules('password', 'Password', 'required');
if ($this->form_validation->run() === FALSE) {
$this->load->view('signin', $data);
} else {
redirect();
}
}
}
/* End of file signin.php */
/* Location: ./application/modules/signin/controllers/signin.php */
Sample code of view signin.php
<?php
defined('BASEPATH') OR exit('No direct script access allowed');
?><!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title><?php echo $title; ?></title>
<style type="text/css">
::selection { background-color: #E13300; color: white; }
::-moz-selection { background-color: #E13300; color: white; }
body {
background-color: #fff;
margin: 40px;
font: 13px/20px normal Helvetica, Arial, sans-serif;
color: #4F5155;
}
a {
color: #003399;
background-color: transparent;
font-weight: normal;
}
h1 {
color: #444;
background-color: transparent;
border-bottom: 1px solid #D0D0D0;
font-size: 19px;
font-weight: normal;
margin: 0 0 14px 0;
padding: 14px 15px 10px 15px;
}
code {
font-family: Consolas, Monaco, Courier New, Courier, monospace;
font-size: 12px;
background-color: #f9f9f9;
border: 1px solid #D0D0D0;
color: #002166;
display: block;
margin: 14px 0 14px 0;
padding: 12px 10px 12px 10px;
}
#body {
margin: 0 15px 0 15px;
}
#container {
margin: 10px;
border: 1px solid #D0D0D0;
box-shadow: 0 0 8px #D0D0D0;
}
</style>
</head>
<body>
<div id="container">
<h1>Signin Here!</h1>
<div id="body">
<?php echo validation_errors(); ?>
<?php echo form_open('signin'); ?>
<p>
<label for="title">Username</label>
<input type="input" name="username" /><br />
</p>
<p>
<label for="text">Password</label>
<input type="password" name="password" /><br />
</p>
<p>
<input type="submit" name="submit" value="Signin" />
</p>
<?php echo form_close(); ?>
<p class="footer">Page rendered in <strong>{elapsed_time}</strong> seconds. <?php echo (ENVIRONMENT === 'development') ? 'CodeIgniter Version <strong>' . CI_VERSION . '</strong>' : '' ?></p>
</div>
</div>
</body>
</html>
After you follow our guideline above, you will get directory structure like screenshot below:
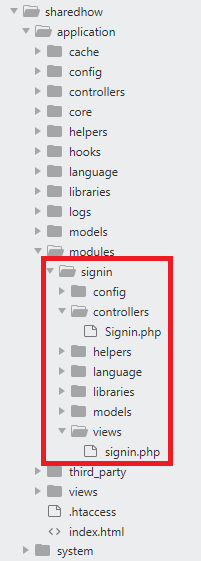
When you run project by web browser, you will get the result like below:
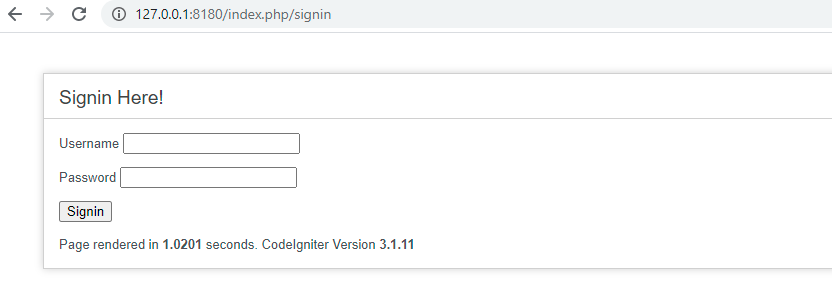
How to move modules folder from application folder
Step 1. Move modules folder to be outside of application folder

Step 2. Create core module for our app. you can use any name depend on your business

Step 3. Open <project root directory>/application/config.php
As we move module from application folder, we need to remove “modules_locations” or comment it.
/*
|--------------------------------------------------------------------------
| Modules locations
|--------------------------------------------------------------------------
|
| These are the folders where your modules are located. You may define an
| absolute path to the location or a relative path starting from the root
| directory.
|
*/
/*$config['modules_locations'] = array(APPPATH . 'modules/');*/
Step 3. Go to application/constants.php
Add below at the bottom of page include_once(APPPATH.’../modules/sharedhow/config/sharedhow_constants.php’);

Step 4. Got to application/routes.php
Add code below at the bottom of page include(APPPATH.’../modules/sharedhow/config/sharedhow_routes.php’)
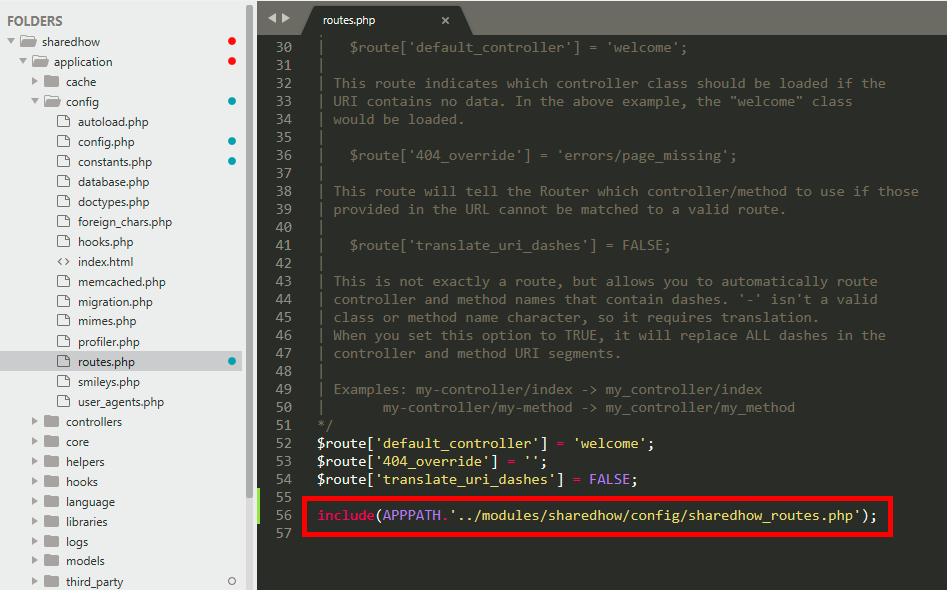
Step 5. Got to application/third_party/MX/Modules.php
Go to line:9 and comment like this //APPPATH . ‘modules/’ => ‘../modules/’, add this code instead : MODULES_PATH => MODULES_FROM_APPCONTROLLERS,

Step 6. Go to modules/sharedhow/config/
Create a file named sharedhow_constants.php and add code below:
<?php
if (!defined('MODULES_FOLDER'))
{
define('MODULES_FOLDER', '../modules');
}
define('HP_FOLDER', 'sharedhow');
define('MODULES_PATH', APPPATH.MODULES_FOLDER.'/');
define('MODULES_FROM_APPCONTROLLERS', '../'.MODULES_FOLDER.'/');
define('HP_PATH', MODULES_PATH.HP_FOLDER.'/');
define('MODULES_WEB_PATH', 'modules/');
# Add code bollow to allow constants modules
// Config isn't loaded yet so do it manually'
include(HP_PATH.'config/sharedhow.php');
foreach ($config['modules_allowed'] as $module)
{
$constants_path = MODULES_PATH . $module . '/config/' . $module . '_constants.php';
if (file_exists($constants_path))
{
require_once($constants_path);
}
}
After you follow step 6, you will get the structure project like screenshot below:

Step 7. Go to modules/sharedhow/config/ and create a file named sharedhow_routes.php and add code below inside the file
<?php
#Add code bellow to allow routes module
// Config isn't loaded yet so do it manually'
include(HP_PATH.'config/sharedhow.php');
$module_folder = MODULES_PATH.'/';
// Load any public routes for advanced modules
foreach ($config['modules_allowed'] as $module)
{
if ($module != HP_FOLDER) // Avoid infinite recursion
{
$routes_path = $module_folder.$module.'/config/'.$module.'_routes.php';
if (file_exists($routes_path)) include($routes_path);
}
}
You will get the structure code like screenshot above after you follow step 7 above:
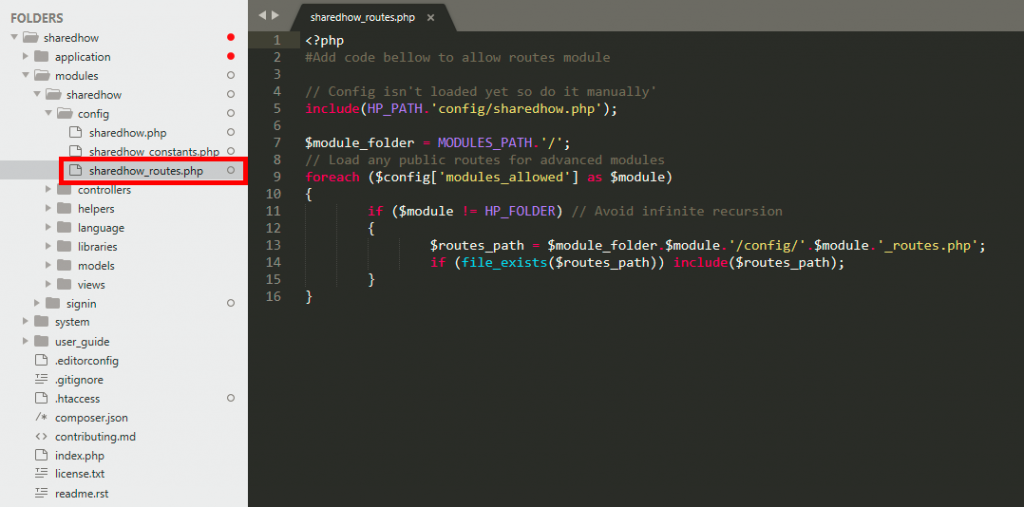
Step 8. It’s last step. Please go to modules/sharedhow/config/ again and create a file named sharedhow.php and add code below inside the file.
$config['modules_allowed'] = array('signin');
Source code
This Post Has 0 Comments